In the Unity engine it’s possible to create you own extension by using editor scripting. An example of this is the custom configurator I created for CP Social.
Modes
To know how editor scripting works you need to know a little bit about how Unity itself works.
When creating a game in Unity you work in the ‘edit mode’ when creating the visual aspect and logic of your game, when testing your game you work in the ‘play mode’ this mode is where Unity runs all your logic for you game.
Both of these modes have so called ‘update loops’, to keep it simple these loops and execute you code over and over again, multiple times a second.
Each mode has their own update loop, if you want more info on the ‘play mode loop’ and the order of execution, you can check the Unity documentation here.
For now we’ll only focus om the edit mode and the ‘Editor Update’.
Editor update
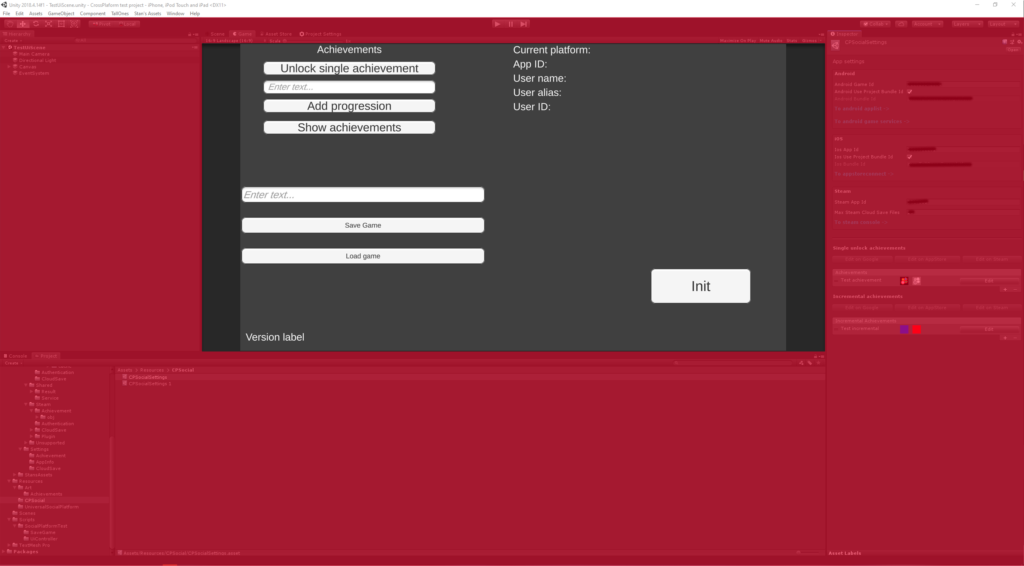
When in the unity editor, the Unity updates the ‘editor update’. This update on a high level only concerns about the editor interface (indicated with a red overlay in the image above).
During this update it checks all interactions made by the user in the editor, button presses, mouse movements, keyboard inputs, etc. This is also where the user interface gets ‘drawn’.
Custom editors
Creating custom editors in Unity is almost the same process as writing game logic in Unity. You have to create a new C# script but instead of the script inheriting from ‘MonoBehaviour’ you have to let it inherit from ‘UnityEditor.Editor’ and add an annotation to the class that describes the type of script (that inherits from MonoBehaviour or ScriptableObject), as show in the image below.
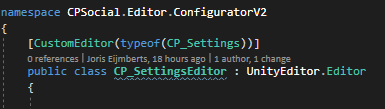
By doing this Unity will recognize your script and will use the logic contained in that script to render a custom editor for you object, in this case the CP_Settings ScriptableObject.
IMGUI
Creating visuals for a custom editor in the Unity game engine make use of a system called IMGUI (Immediate Mode GUI), this is a type of GUI (graphical user interface) that does not retain any information about itself eg. it doesn’t store any data about it’s state.
This type of GUI is heavily code driven and not very optimized for in-game use. But for creating editor windows it’s very well suited, so by default all editor windows in Unity are made with this system.
For more info on IMGUI, read this detailed blog by Unity.
UIElements
As of Unity 2018.3, it’s possible to create editor windows using UIElements an xml based approach to creating editor window, for more info read more about it in the Unity documentation, Unity UIElements…
I didn’t use Unity UIElements for the CP Social configurator, seen as no-one at The Tall Ones had ever used it before and were more comfortable with using the IMGUI approach.
Update
As described in the previous paragraph ‘editor update’ the editor uses an update loop to update all opened editor. During this update it check’s for user interactions.
To capture these interactions and add you own custom editor logic you have to override a method in UnityEditor.Editor called, OnInspectorGui(). By doing this all the logic in that method will be execute every time the editor updates.
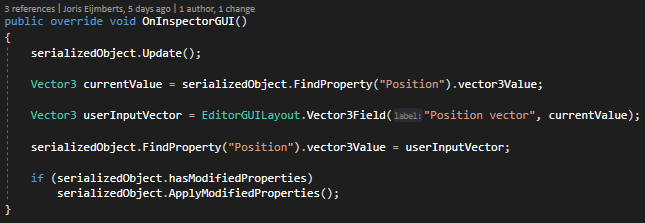
The image above shows the final OnInspectorGui() method of the example show below.
SerializedObject & SerializedProperty
When creating a custom editor in Unity it’s important to keep in mind that actions performed in the editor need to be ‘undo-able’, this is where SerializedObject and SerializedProperty come in play, these two object give developer a generic way to access serialized fields in Unity objects.
When using the SerializedObject and SerializedProperty, you get to access and edit serialized fields, these edits made will be automatically detected by the Unity editor and when applied be automatically saved and undo-able.
Example
The image below shows how a SerializedObject can use a SerializedProperty be used retrieve a Vector3 value from a field called Position.
It also show how you can draw an editor field so that the user can edit the value, drawing the editor field is done using ‘EditorGUILayout.Vector3Field‘
The last part where the ‘hasModifiedProperties‘ ‘if’-statment is called, it where the check is done if the SerializedObject has any modification done to it. If so that using the ‘ApplyModifiedProperties’ method these modifications are applied to the ‘SerializedObject‘ and saved with an undo action.
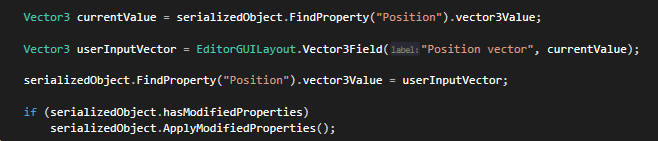
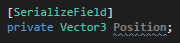

CP Social configurator
The CP Social configurator is a custom editor created using the techniques shown on this page. It’s a custom editor created for a SciptableObject containing all CP Social’s settings.
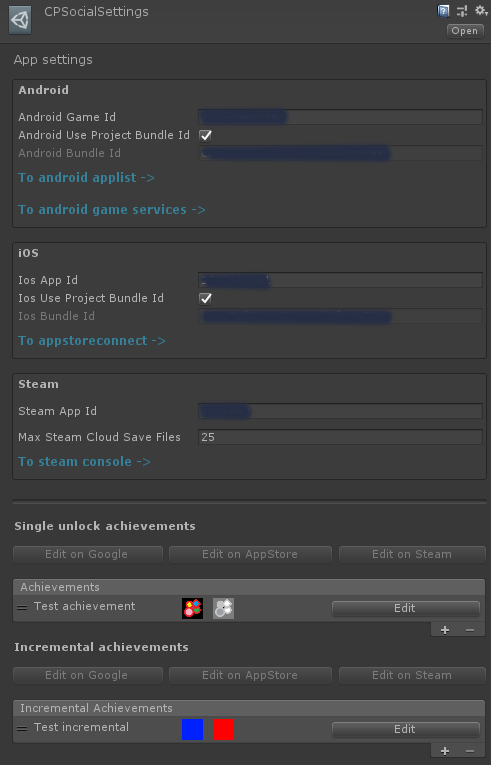